12-Factor Apps in Kubernetes: A Comprehensive Guide
The Twelve-Factor App methodology, originally proposed by Heroku, provides a set of best practices for building scalable, maintainable, and cloud-native applications. Over time, these principles have been adapted by organizations like F5 and IBM to fit modern microservices architectures and Kubernetes environments.
At Retesys, we specialize in architecting and developing cloud-native solutions based on Kubernetes, leveraging these best practices to ensure scalability, resilience, and maintainability.
This guide combines Heroku's original 12-Factor App guidelines with updated recommendations from F5, IBM, and modern development practices to help you implement these principles effectively in Kubernetes environments.
1. Codebase
-
Concept:
The codebase factor ensures that there is only one codebase per application, managed through a version control system, to maintain consistency and traceability across environments.
-
Best Practice:
Use one codebase per microservice, managed with a version control system like Git. If code needs to be shared, use libraries or packages. Adopt GitOps methodologies and Infrastructure as Code (IaC) approaches by storing configuration files for CI/CD tools (e.g., Jenkins pipelines, Argo CD configurations) in Git.
-
Modern Approach:
Utilize GitOps tools such as Argo CD and Flux for automated deployment and environment management directly from Git repositories. Store Helm charts and CI/CD configuration files in version-controlled repositories. Use branching strategies to manage different environments like development, staging, and production.
2. Dependencies
-
Concept:
Explicit declaration and isolation of dependencies ensure that the application does not rely on any implicit system-wide packages and can be consistently built across different environments.
-
Best Practice:
Explicitly declare and isolate dependencies using package management tools like Npm, NuGet, Maven, or Gradle. Use private and public package registries (e.g., JFrog Artifactory, Nexus, MS Azure Artifacts). Generate a Software Bill of Materials (SBOM) for each application or library for security purposes.
-
Modern Approach:
Incorporate dependencies directly into container images during the build process, ensuring that all required libraries and tools are present at runtime. Automate dependency checks and SBOM generation using CI/CD pipelines to detect vulnerabilities early. Use Kubernetes-native tools to manage container dependencies and isolate environments effectively.
3. Config
-
Concept:
Configuration should be stored separately from the codebase, using environment variables or configuration management tools, to facilitate environment-specific adjustments without altering the code.
-
Best Practice:
Store configuration data in Kubernetes ConfigMaps and Secrets. Use externally managed configuration management tools like Spring Cloud Config Server, and for secrets, use HashiCorp Vault. Employ Vault Agent Injector to inject secrets into pods as files or environment variables without embedding client libraries in application code.
-
Modern Approach:
Integrate HashiCorp Vault with Kubernetes for dynamic secrets management and encryption at rest. Utilize ConfigMaps for non-sensitive configuration data and Secrets for sensitive information. Automate configuration updates across environments using GitOps pipelines and Kubernetes ConfigMap reloading mechanisms.
4. Backing Services
-
Concept:
Treat all backing services (e.g., databases, message brokers) as attached resources, ensuring they can be easily swapped or moved without impacting the application code.
-
Best Practice:
Backing services can run inside Kubernetes in pods or outside of Kubernetes. Use Kubernetes’ built-in service discovery for internal services and consider service discovery tools like HashiCorp Consul or Netflix Eureka for more complex setups.
-
Modern Approach:
Use Kubernetes DNS and service discovery features for internal communication between services. For external service discovery, use HashiCorp Consul or Netflix Eureka. Consider using Steeltoe library in .NET environments, which provides clients for both Consul and Eureka along with other useful tools for managing microservices.
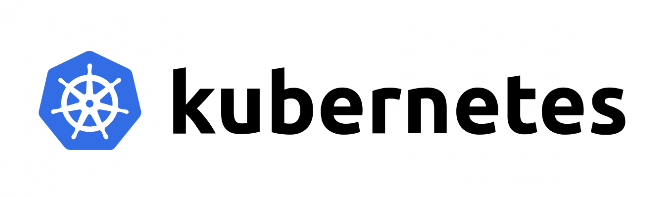
5. Build, Release, Run
-
Concept:
Separating the build, release, and run stages helps maintain a clear distinction between different parts of the application lifecycle, enhancing manageability and stability.
-
Best Practice:
Prefer using Helm charts because Helm charts are better aligned with the "Build, Release, Run" principle of the 12-factor methodology for several reasons:
-
Separation of Concerns:
Helm clearly separates build (container image creation), release (packaging of code and configuration into charts), and run (deployment of charts into a Kubernetes cluster).
-
Versioning and Release Management:
Helm provides built-in support for managing versions of the application and configuration, enabling consistent and traceable deployments. This aligns with the need to have repeatable releases and the ability to easily roll back to previous versions.
-
Consistency and Reusability:
Helm's templating and packaging system help ensure that deployments are consistent across environments (development, staging, production) and make it easier to manage complex configurations.
-
Deployment and Rollback:
Helm facilitates not just initial deployments but also upgrades and rollbacks, which are essential aspects of managing the run phase in a consistent and predictable manner.
Learn more about using Helm for Kubernetes deployments on the official Helm website.
-
Separation of Concerns:
-
Modern Approach:
Use CI/CD tools like Jenkins, Argo CD, GitLab CI, and Spinnaker to automate the build, release, and run stages, ensuring that container images are built, tested, and pushed to container registries. Automate Helm chart deployment through CI/CD pipelines for consistent and repeatable deployments.
For more on deploying Linux containers, see our article on Top 2 CI/CD Tools and Kubernetes Deployments.
6. Processes
-
Concept:
Processes should be stateless and share-nothing, ensuring that each instance can run independently, scale horizontally, and be replaced if necessary.
-
Best Practice:
Design microservices to be stateless. Use Kubernetes Deployments for stateless services and StatefulSets for stateful components. Employ service brokers and event-streaming systems like RabbitMQ and Apache Kafka for asynchronous communication.
-
Modern Approach:
Ensure that each microservice handles only its specific functionality, promoting single responsibility design. Use Apache Kafka for building event-driven architectures, which facilitate decoupled communication between services. Manage state externally using stateful services or databases, ensuring that stateless microservices can be scaled and redeployed without issues.
7. Data Isolation
The Single Responsibility Principle (SRP):
Gather together those things that change for the same reasons and at the same times. Separate those things that change for different reasons or at different times.
Robert C. Martin
-
Concept:
Each microservice should manage its own data storage to ensure data integrity and avoid tight coupling between services. Data isolation ensures that microservices can function independently, improving reliability and scalability.
-
Best Practice:
Use Kubernetes Persistent Volumes and StatefulSets for managing stateful data. Implement data replication and sharding for scalability and resilience, especially in high-availability microservices.
The concept of data isolation in microservices architecture, where each microservice manages its own data storage, aligns closely with Domain-Driven Design (DDD) and the Single Responsibility Principle (SRP). DDD structures software around specific business domains, ensuring each microservice encapsulates its domain's functionality and data, promoting loose coupling.
This approach allows services to evolve independently and aligns with SRP by limiting each microservice to its own logic and data, reducing the risk of unintended side effects. Data isolation enhances scalability, maintainability, and system robustness, enabling teams to innovate and scale services without disrupting others, thus providing greater flexibility and adaptability to changing business needs.
-
Network Isolation and Security:
All pods can talk to each other in the network by default. In certain industries, enhanced security is required at the network level, such as in multi-tenant SaaS environments or software that needs to comply with regulations like HIPAA, SOC 2, or PCI. In addition to data isolation, network microsegmentation can be implemented using Kubernetes Container Network Interface (CNI) plugins like Tigera Calico.
A CNI networking plugin can enforce network isolation by applying networking policies for defined security domains. This approach ensures that if one tenant or application is compromised, the likelihood of a malicious actor gaining access to sensitive information from other tenants is significantly reduced.
For best practices on securing Kubernetes clusters, read our guide on Enhanced Kubernetes Security.
8. Concurrency
-
Concept:
Efficient handling of multiple concurrent processes is essential for scalability and responsiveness in microservices architecture.
-
Best Practice:
Use Kubernetes Horizontal Pod Autoscaler (HPA) and Vertical Pod Autoscaler (VPA) to automatically scale microservices based on metrics like CPU and memory usage.
-
Modern Approach:
Implement HPA to scale the number of pod replicas dynamically based on load. Use VPA to adjust resource requests and limits automatically, optimizing resource utilization. Utilize event-driven architecture and reactive programming frameworks (e.g., Spring WebFlux) to manage high-concurrency scenarios effectively.
9. Disposability
-
Concept:
Services should start and stop quickly to facilitate elastic scaling, rapid deployment, and robust fault tolerance.
-
Best Practice:
Use Kubernetes readiness and liveness probes to manage container lifecycles effectively. Implement resilience patterns like Circuit Breaker, Retry, and Timeout to handle transient faults in communication between microservices.
-
Modern Approach:
Define readiness and liveness probes in Kubernetes deployments to ensure services are only routed traffic when ready and can self-heal if issues are detected. Use resilience libraries like Polly (for .NET) and Hystrix (for Java) to implement patterns that enhance fault tolerance and improve overall system robustness.
10. Dev/Prod Parity
-
Concept:
Keeping development, staging, and production environments as similar as possible helps reduce bugs and configuration drift.
-
Best Practice:
Use the same container images built by CI/CD tools across different environments (development, staging, production) to maintain consistency.
-
Modern Approach:
Store and version container images in a centralized container registry to ensure consistency across environments. Automate the deployment process using GitOps practices to promote the same image through different stages, maintaining parity and reducing configuration drift.
11. Logs
-
Concept:
Treat logs as event streams, allowing for centralized management and analysis to monitor the health and performance of microservices.
-
Best Practice:
Use logging and monitoring tools like Prometheus and the ELK stack (Elasticsearch, Logstash, Kibana). Implement distributed tracing systems like Zipkin or Jaeger to track requests across microservices. Assign correlation IDs to simplify tracing and ensure consistency in log entries.
-
Modern Approach:
Set up centralized logging using the ELK or EFK (Fluentd instead of Logstash) stack to aggregate and analyze logs from various microservices. Implement distributed tracing using Jaeger or Zipkin to visualize and trace request flows across services. Use Prometheus for real-time monitoring and alerting based on custom metrics.
12. Admin Processes
-
Concept:
Run administrative and management tasks as one-off processes that do not interfere with the main application processes.
-
Best Practice:
Use Kubernetes Jobs for executing one-off administrative tasks and CronJobs for scheduled maintenance tasks.
-
Modern Approach:
Leverage Kubernetes Jobs for tasks like database migrations or batch processing that need to run once. Use CronJobs for recurring tasks such as cleaning up temporary files or generating periodic reports. Automate the deployment and monitoring of these jobs using CI/CD tools integrated with GitOps workflows.
Conclusion
Adopting the 12-Factor App methodology in a Kubernetes environment ensures that microservices are scalable, maintainable, and resilient. By following these updated best practices, teams can leverage Kubernetes' native capabilities and integrate modern tools and frameworks to build robust cloud-native applications. Whether using Helm for deployment, managing configurations with HashiCorp Vault, or ensuring service resilience with tools like Polly and Hystrix, these strategies provide a solid foundation for building and operating microservices effectively in a Kubernetes landscape.
At Retesys, our cloud software experts can help you implement modern 12-factor app principles, migrate legacy systems to the cloud, and build scalable, cloud-native applications.
Contact us today to learn how we can streamline your cloud migration journey and ensure your infrastructure is ready for the future.
Contact Us